TCP #8: How a 17th-century shipbuilding technique is revolutionizing modern software.
Keeping your software's engine room safe from disaster.
You can also read my newsletters from the Substack mobile app and get notified when a new newsletter issue comes out.
Did you know a single failure can take down your entire digital infrastructure if not properly isolated?
You wouldn’t want your entire application to crash just because one service is overwhelmed, would you?
That’s where the Bulkhead Design Pattern comes into play. It sections off your services, ensuring that the failure of one doesn’t affect the rest.
In today’s newsletter, I will cover:
Overview
Need for Resilience in Microservices
What is the Bulkhead Design Pattern?
Implementing the Bulkhead Design Pattern
Benefits
Challenges and considerations
Let’s dive in.
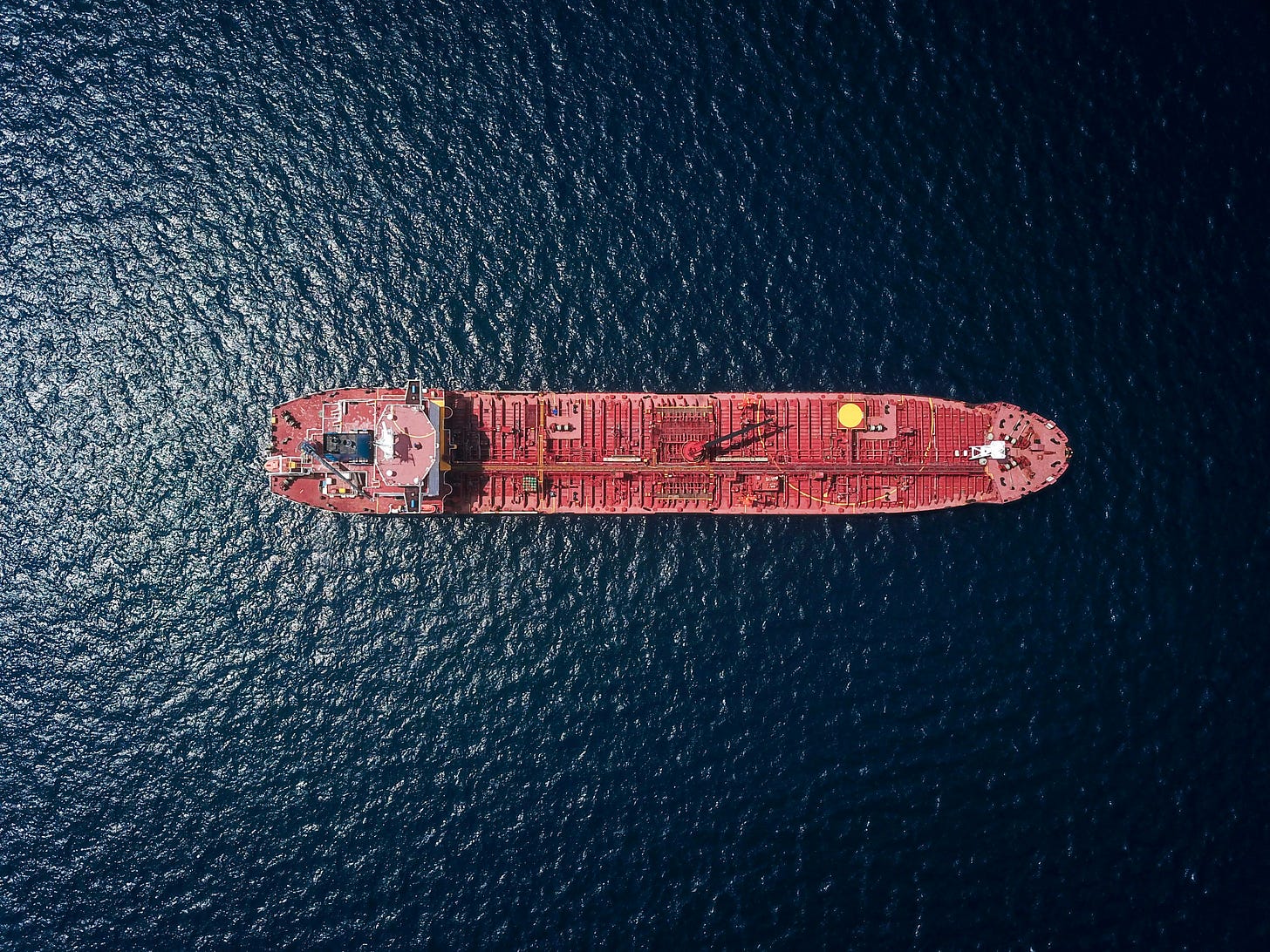
Overview
The term "Bulkhead" originated from shipbuilding. In ships, bulkheads are internal walls that create watertight compartments, which can contain flooding in one compartment without letting it spread to others.
This principle of compartmentalization is adopted in software design to prevent failures in one part of a system from propagating to the entire system.
In software architecture, the Bulkhead design pattern creates isolated segments within an application where each segment can operate independently.
If one segment fails due to overloading or a crash, the others function normally.
This pattern is especially relevant in microservices architecture, where different services cater to different functionalities of an application.
Need for Resilience in Microservices
Resilience is the ability of a system to handle and recover from failures gracefully.
In a microservices architecture, resilience is particularly crucial because the architecture inherently involves multiple services communicating over a network.
This complexity can introduce several points of failure that need to be managed effectively.
Importance of Resilience
In a distributed system like microservices, components can fail due to various reasons such as network issues, hardware failures, or spikes in traffic.
If a system is not resilient, a failure in one component can lead to a cascade of failures throughout the system, potentially bringing down the entire application.
Resilient systems are designed to detect failures quickly, prevent them from spreading, and recover without significant downtime.
Examples of Potential Failures
Service Downtime: Individual services may become unavailable due to software bugs, resource limits, or infrastructure problems. Resilient systems ensure that such downtimes affect minimal application parts and have fallbacks or redundancies in place.
Network Failures: Network issues can prevent services from communicating effectively. Resilience in this context means having strategies like retries, timeouts, and circuit breakers to manage these interruptions.
Resource Exhaustion: Services might face issues like memory leaks or excessive CPU usage under high load. Resilient systems use patterns such as Bulkheads to isolate resource usage and prevent one service’s issues from affecting others.
What is the Bulkhead Design Pattern?
The Bulkhead pattern involves segregating application elements into pools so that if one fails, the others will continue to function.
For example, if a service for processing payments is overwhelmed, it won’t affect the service handling customer inquiries.
Implementation Techniques
Thread pools: Assign specific numbers of threads to different services. New requests are queued or rejected if a service reaches its limit, but other services' threads remain unaffected.
Semaphore: Use semaphores to limit the number of concurrent requests a service can handle. Once the limit is reached, additional requests are either queued or rejected, protecting other services from overloading.
Resource isolation: This could mean running services on separate servers, virtual machines, or containers to isolate resources physically.
Types of Isolation
Thread-Level: This approach limits the number of threads allocated to each service, ensuring that a failure or deadlock in one thread pool does not impact others.
Semaphore-Level: Semaphores control access to shared resources, such as databases or external APIs, by limiting the number of concurrent service requests, preventing system overloads.
Process-Level: Services run in entirely separate processes, possibly on different hardware, offering the highest level of isolation and resilience but at the cost of increased resource usage and complexity.
The Bulkhead pattern's effectiveness lies in its ability to localize problems and maintain system availability despite individual service failures. This makes it an essential pattern for building robust microservices architectures where uptime and reliability are critical.
Implementing the Bulkhead Design Pattern
Implementing the Bulkhead pattern in microservices is about ensuring that the system can withstand and isolate failures effectively.
The following guide can help:
Identify Services for Isolation: Start by identifying critical services that, if failed, could severely impact the performance or availability of your application. These are your primary candidates for applying the Bulkhead pattern.
Define Resource Limits: For each selected service, define limits on the resources it can use. These limits could be regarding the number of concurrent threads, processes, or network connections.
Implement Isolation Mechanisms:
Thread Pools: Configure separate thread pools for each service. This way, if one service's thread pool is exhausted, it won’t affect other services.
Semaphores: Use semaphores to limit the number of concurrent requests a service can handle at any time.
Containerization: Deploy services in separate containers or virtual machines to achieve physical isolation.
Test Failure Scenarios: Simulate different failure scenarios to ensure effective isolation. Check how the system behaves when one service maxes out its resource limits and ensure that other services operate smoothly.
Monitor and Adjust: Once implemented, continuously monitor the system’s performance. Be prepared to adjust resource limits and isolation settings based on real usage patterns and system load.
Tools and Technologies
Libraries and Frameworks: Libraries like Hystrix or Resilience4j provide built-in support for implementing Bulkhead and other resilience patterns. These libraries offer features like thread and semaphore isolation out of the box.
Containerization Tools: Docker and Kubernetes can facilitate process-level isolation by allowing each service to run in its container or pod, thus ensuring that the failure of one container does not affect others.
Monitoring Tools: Tools like Prometheus or Grafana are critical for monitoring services' health and resource usage, helping to ensure that Bulkhead settings are correctly calibrated.
Here is a simple example of setting up a semaphore-based Bulkhead using Java:
import java.util.concurrent.Semaphore;
public class ServiceWithBulkhead {
private static final int MAX_CONCURRENT_REQUESTS = 5;
private final Semaphore semaphore = new Semaphore(MAX_CONCURRENT_REQUESTS);
public void serviceMethod() {
try {
semaphore.acquire();
// Code for the service logic here
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
semaphore.release();
}
}
}
In this example, the ServiceWithBulkhead class uses a semaphore to limit itself to a maximum of five concurrent requests, effectively isolating its load from other system parts.
Benefits
Implementing the Bulkhead pattern in microservices architecture provides several important benefits that enhance system stability and reliability.
These advantages are crucial for maintaining high service availability and ensuring a robust user experience.
Improved System Stability
Isolation of Failures: The core benefit of the Bulkhead pattern is its ability to isolate failures within a single service or a group of services. By preventing a single point of failure from affecting the entire system, Bulkhead ensures that the rest of the application remains operational even if one part becomes overloaded or fails.
Prevention of Cascading Failures: The Bulkhead pattern prevents failures from cascading across the system by limiting their impact to isolated areas. This containment is essential in a distributed environment where services depend on each other.
Enhanced Fault Tolerance
Graceful Degradation: In the event of a service failure, the Bulkhead pattern allows the application to continue functioning in a degraded mode. For example, if the service handling payment processing is down, the application can still serve browsing and cart functionality.
Managed Resource Utilization: Bulkheads help manage resource utilization by capping the maximum resources (like threads, CPU, memory) that a service can consume. This management prevents any single service from exhausting system resources, thereby maintaining overall system performance.
Challenges and Considerations
While the Bulkhead pattern significantly enhances the resilience of microservices architectures, its implementation has challenges and considerations.
Common Pitfalls
Increased Complexity: Each service or resource may need individual configuration and management for isolation, which can complicate the architecture and deployment processes.
Resource Inefficiency: Allocating separate resources (like threads or connection pools) to different services can lead to underutilization. If not carefully managed, this could result in an inefficient use of system resources, as isolated pools may remain idle while other services are resource-starved.
Overhead Concerns
Performance Impact: Introducing isolation mechanisms such as thread pools and semaphores can add latency to service responses. The additional overhead of managing these mechanisms must be balanced against their benefits in preventing service failures.
Monitoring and Management: With the implementation of Bulkhead, monitoring becomes more critical and potentially more demanding. You'll need to continuously observe isolated segments' performance and resource utilization to adjust configurations and prevent bottlenecks.
Balancing Isolation with Complexity
Right-Sizing Isolation: It's essential to correctly size the isolation levels for each service based on their criticality and resource demands. Over-isolating can lead to resource wastage while under-isolating can fail to prevent cascading failures.
Dynamic Resource Allocation: Implementing dynamic resource allocation mechanisms can help mitigate some inefficiencies associated with static resource limits. Techniques such as autoscaling and adaptive thresholds can help adjust resources based on actual demand in real-time.
Holistic Approach to Resilience: Bulkhead should be part of a broader resilience strategy that includes other patterns, such as circuit breakers and retries. This integrated approach helps manage different types of failures more effectively.
Key Takeaways
The Bulkhead pattern's core philosophy, preventing failures in one part of the system from impacting the entire system, mirrors the pragmatic approach in naval architecture, where bulkheads compartmentalize a ship to contain damage.
Isolation is Key: The Bulkhead pattern enhances system stability by isolating failures to individual services or resources. This isolation helps maintain overall system functionality even when parts of it fail.
Improves Fault Tolerance: By compartmentalizing services, the Bulkhead pattern allows the system to operate under partial failure, achieving a level of fault tolerance crucial for maintaining service availability and reliability.
Complements Other Patterns: While powerful, the Bulkhead pattern often works best with other resilience patterns, such as Circuit Breakers and Retry mechanisms. This combination helps address a wide range of failure scenarios.
Implementation Considerations: Applying the Bulkhead pattern involves thoughtful decisions about resource allocation and system design. It requires balancing between complexity and resilience benefits to avoid unnecessary overhead.
As we continue to build and evolve our software architectures, integrating patterns like Bulkhead enhances the robustness of our systems and ensures that we can deliver continuous service to users, even in the face of failures.
If you have any observations or views about this post, please leave a comment.
Shoutout
5 Strategies for High-Availability Systems by
: Saurabh discusses 5 strategies to make your system highly available.Caching: the single most helpful strategy for improving app performances by
: Deep dive by Dr. Milan on the importance and types of caching to improve app performance.How Lyft supports rides to 21 Million users by
: Deep dive on Lyft’s architecture and engineering and how it provides its key features to a growing user base
That’s it for today!
Did you enjoy this newsletter issue?
Share with your friends, colleagues, and your favorite social media platform.
Until next week — Amrut
Whenever you’re ready, there are 2 ways I can help you:
Are you thinking about getting certified as a Google Cloud Digital Leader? Here’s a link to my Udemy course, which has helped 569+ students prepare and pass the exam. Currently, rated 4.68/5. (link)
Course Recommendation: AWS Courses by Adrian Cantrill (Certified + Job Ready):
ALL THE THINGS Bundle (I got this. Highly recommend it!)
Note: These are affiliate links.
Get in touch
You can find me on LinkedIn or X.
If you wish to request a topic you would like to read, you can contact me directly via LinkedIn or X.